Handling Events
Catching run-time errors is helpful but it is best practice to prevent errors from occurring in the first place. The previous error can be prevented by disabling the Move button while a motion is in progress. This functionality is implemented in three steps:
- Disable the Move button after the user click
- Enable reporting of a completed motion.
- Enable the Move button once the motion is complete.
Disable the Move Button after a User Click
In the code view, add the highlighted code below to the MoveButton_Click subroutine:
Private Sub MoveButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MoveButton.Click
MoveButton.Enabled = False
Try
Controller.Motion(0).Trapezoidal(Target.Text)
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
This code will disable the Move button when a move is commanded.
Enable Event Reporting
The MPX control allows the controller to notify you of events generated by the Motion Object. In the code view, add the highlighted code below to Form1_Load to enable the notification of a MotionDone event.
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Try
Controller.Motion(0).AxesClear()
Controller.Motion(0).AxisAdd(0)
Controller.Motion(0).DefaultSpeed = 1000
Controller.Motion(0).DefaultAccel = 10000
Controller.Motion(0).DefaultDecel = 10000
Controller.Motion(0).EventEnable(MPX.EventType.MotionDone)=True
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
Enable the Move Button after Motion is Complete
Now that event reporting of a MotionDone event is enabled, you must add a subroutine that checks for the MotionDone event from Motion Object 0 and enables the Move button if the MotionDone event is received.
Return to the Form1.vb [Design] tab and double-click on the MPX Controller icon to generate the Controller_ControllerEvent subroutine.
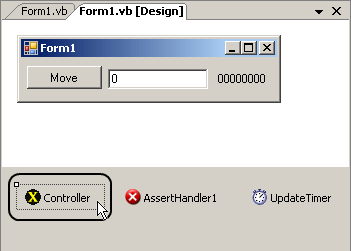
The cursor will now be placed in a subroutine called Controller_ControllerEvent:
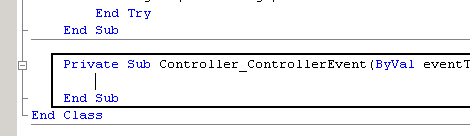
Add the highlighted code below to the Controller_ControllerEvent subroutine to check for the MotionDone event and enable the Move button if the event is received:
Private Sub Controller_ControllerEvent(ByVal eventType As MPX.EventType, _ByVal objectNumber As System.Int32, ByVal int32Info() As System.Int32, _ByVal int64Info() As System.Int64) Handles Controller.ControllerEvent
Try
If (eventType = MPX.EventType.MotionDone) And (objectNumber = 0) Then
MpxUtil.Control.Enable(MoveButton)
End If
Catch exc As System.Exception
MsgBox(exc.ToString())
End Try
End Sub
Code Details
There are several details to note in this code:
- The entire subroutine is wrapped in a try-catch statement. The catch statement catches all exceptions. This is to prevent any exception that is thrown in Controller_ControllerEvent from being caught in the MPX event distributor thread. If an exception did propagate to the event distributor, it would be ignored, but this would not be very useful for the developer.
- The MoveButton control is not enabled directly. Rather, it is enabled by calling MpxUtil.Control.Enable. Setting MoveButton.Enable directly from within the Controller_ControllerEvent subroutine will cause an exception to be thrown because it is illegal to access Windows forms or controls from any thread other than the thread in which they were created: usually, the main thread. MpxUtil.Control.Enable uses a well-documented technique for accessing controls from the thread in which they were created. The source for MpxUtil is distributed with the MPX.
Previous | Next |