Handling Errors
In this section, you will cause the application to throw an exception (generate a run-time error) by performing an invalid operation. You will then use Visual Basic’s error handling mechanism to handle the error.
Generate the Error
Run the sample application and move the axis to position 5000. When the motion is complete, enter 0 in the text box and click on the Move button twice. The second click commands another motion while the first motion is still being executed, causing an exception to be thrown. Code execution is halted and you are returned to the code view.
A dialog box may appear prompting you for the location of the MPX source file where the exception was thrown. If so, click Cancel to close the dialog box.
When an exception is thrown, the application terminates and Visual Basic displays a dialog box describing the error (shown below) from which the application cannot recover and highlights the line of code from which the exception was thrown. Now that you know where the exception occurred, you can make the necessary changes to the code to handle them. Close this window to return to the Visual Basic development environment.
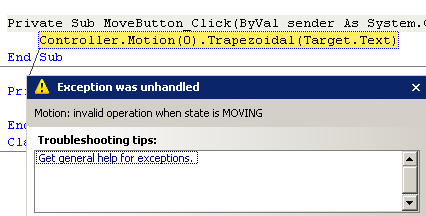
Handling the Exception with a Try-Catch Statement
In a real application, you would not want your application to terminate if an error occurred. In this example, Visual Basic’s structured exception handling will be used to handle the error.
Click on the Form1.vb tab to return to the code view. Wrap a Try-Catch statement around the original code in the MoveButton_Click subroutine, as shown below:
Private Sub MoveButton_Click(ByVal sender As System.Object, _ByVal e As System.EventArgs) Handles MoveButton.Click
Try
Controller.Motion(0).Trapezoidal(Target.Text)
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
The Try statement specifies that the trapezoidal move is to be tested for exceptions. If an exception is thrown, the Catch statement catches an MPX exception and displays a message box with information about the exception (shown on the right).
Run the application and generate the same error as before. When the exception is thrown, the message box shown on the right will be displayed to notify you of the error. Clicking OK will return you to the running application.
To help ensure the reliability of your application, wrap all MPX code with Try-Catch statements as shown below:
Public Class Form1
Private Sub Form1_Load(ByVal sender As System.Object,_
ByVal e As System.EventArgs) Handles MyBase.Load
Try
Controller.Motion(0).AxesClear()
Controller.Motion(0).AxisAdd(0)
Controller.Motion(0).DefaultSpeed = 1000
Controller.Motion(0).DefaultAccel = 10000
Controller.Motion(0).DefaultDecel = 10000
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
Private Sub MoveButton_Click(ByVal sender As System.Object, _ByVal e As System.EventArgs) Handles MoveButton.Click
Try
Controller.Motion(0).Trapezoidal(Target.Text)
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
Private Sub UpdateTimer_Tick(ByVal sender As System.Object, _ByVal e As System.EventArgs) Handles UpdateTimer.Tick
Try
ActualPosition.Text = Controller.Axis(0).ActualPosition
Catch exc As MPX.Exception
MsgBox(exc.Message)
End Try
End Sub
End Class
With these Try-Catch statements added, any exceptions or errors that might occur during the execution of MPX code will be caught and displayed in a message box, but your application will continue running.
Previous | Next |