Demand Mode Switching
Overview
Suppose the application needs to move one or more axes to a location, switch from closed-loop position control to torque control (SynqNet velocity demand mode to torque demand mode), limit the maximum force, then switch back to position control (SynqNet velocity demand mode) and move to a second location.
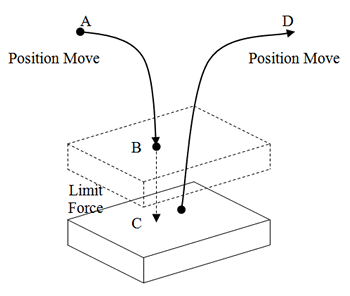
Example #1 - Single filter object with manual switching
Position |
Description |
A to B |
Move component from position A to B using closed-loop position control (velocity demand mode). |
B |
User application changes controller closed-loop parameters using the gain table index and switches from velocity demand mode to torque demand mode. The torque would be limited by the filter gain table 1's high and low output limits. |
B to C |
Move to position C (torque is limited by gain table 1), wait for settling, and release the part. |
C to D |
Start move to position D. Switch back to gain table 0 and switch from torque demand mode to velocity demand mode. |
MPI
The example code fragment below shows how to use the gain table index and demand mode switching. It does not include the steps to configure the gain table parameters. For details about gain tables, see Gain Tables.
NOTE: This code fragment does not contain return value checking or all the necessary logic for a real application. It is for demonstration purposes only.
|
/* move to B */
mpiMotionSimpleTrapezoidalMove(motion,
positionB,
velocity,
acceleration,
deceleration);
/* wait for motion to settle using events */
mpiNotifyEventWait(notify, &eventStatus, MPIWaitFOREVER);
if (eventStatus.type == MPIEventTypeMOTION_DONE) {
/* switch filter params for TORQUE mode */
mpiFilterGainIndexSet(filter, 1); /* gain table 1 */
/* switch to torque mode */
mpiMotorDemandModeSet(motor, MPIMotorDemandModeTORQUE);
mpiMotionSimpleTrapezoidalMove(motion,
positionC,
velocity,
acceleration,
deceleration);
}
/* wait for motion to settle using events */
mpiNotifyEventWait(notify, &eventStatus, MPIWaitFOREVER);
if (eventStatus.type == MPIEventTypeMOTION_DONE) {
/* move to D */
mpiMotionSimpleTrapezoidalMove(motion,
positionD,
velocity,
acceleration,
deceleration);
/* switch filter params for VELOCITY mode */
mpiFilterGainIndexSet(filter, 0); /* gain table 0 */
/* switch to VELOCITY mode */
mpiMotorDemandModeSet(motor, MPIMotorDemandModeVELOCITY);
}
|
MPX
Not supported.
Example #2 - Dual-filter objects with automatic switching
The user application configures two controller filter objects. The velocity mode filter object is configured with closed-loop control parameters. The torque mode filter object is configured with closed-loop control parameters and high/low output limits.
Position |
Description |
A to B |
Move component from position A to C using closed-loop position control (velocity demand mode). |
B |
At position B, switch from velocity demand mode to torque demand mode with a position triggered user limit. The closed-loop control shall switch from the velocity mode filter object to the torque mode filter object. |
B to C |
Wait for settling and release the component. |
C to D |
Start move to position D. At position B, switch from torque demand mode to velocity demand mode with a position triggered user limit. The closed-loop control shall switch from the torque mode filter object to the velocity mode filter object. |
MPI
See the mode_switch.c sample application for an example implementation of dual-filter objects with automatic switching.
MPX
Not supported.
See Also
Gain Tables | SynqNet Demand Modes
|